| |
|
AnalogMeter
The GUI component AnalogMeter graphically displays an analogue gauge. Its size is freely scaleable and an optional caption may be added. Furthermore, the opening angle and orientation of the scale, as well as the amount of scale lines can be adjusted. Table 1 summerises the most important methods of AnalogMeter :
|
Method | Description |
AnalogMeter(int x, int y, int width, int height, int min, int max, int openAngle, int orientation, int dials) | Creates a new AnalogMeter at the specified coordinates. The parameters min and max specify the range of values, dials represents the amount of scale lines. openAngle is the desired maximal hand deflection and orientation adjusts the gauges' orientation (possible values are ORIENTATION_CENTER , ORIENTATION_LEFT and ORIENTATION_RIGHT ). If orientation equals ORIENTATION_LEFT or ORIENTATION_RIGHT , the maximal hand deflection (openAngle ) is limited to 90°. |
setCaption(String captionMin, String captionMax) | Adds a caption to the AnalogMeter . The passed Strings will be displayed at the beginning and at the end of the scale. |
setNumericDisplay(int digits, int decimals, String unit) | This method configures the alphanumeric display of measured values. Parameter digits defines the total number of displayed digits, decimals specifies the number of decimals included in digits . Example: digits=4 and decimals=3 will display values in form of x,xxx . If decimals=0 , no decimal point will be displayed (useful if the measured value shall be displayed in a different range of values). The parameter unit holds the unit of the displayed values (e.g. "V"). |
setValue(int value) | Passes a new value to the AnalogMeter , which thereupon will be updated. If value exceeds the range of values, it will be replaced by the according value for min or max . |
Table 1: Methods of class jcontrol.ui.vole.meter.AnalogMeter
The program example VoleAnalogMeterExample listed below demonstrates the usage of the AnalogMeter and some of its methods listed in table 1. It is included in the ZIP archive VoleAnalogMeterExample.zip along with the homonymous JControl/IDE project file. Use the simulator or your JControl device to see the program running.
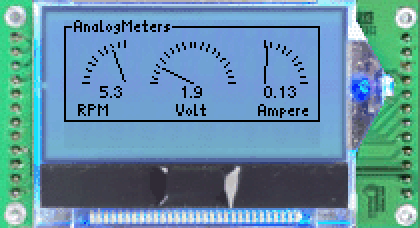
Figure 1: The VoleAnalogMeterExample
1 | import jcontrol.lang.Math; |
2 | import jcontrol.lang.ThreadExt; |
3 | import jcontrol.ui.vole.Border; |
4 | import jcontrol.ui.vole.Container; |
5 | import jcontrol.ui.vole.Frame; |
6 | import jcontrol.ui.vole.Label; |
7 | import jcontrol.ui.vole.meter.AnalogMeter; |
8 | |
9 | /** |
10 | * <p>This example demonstrates how to use the |
11 | * component AnalogMeter within the GUI framework |
12 | * JControl/Vole.</p> |
13 | * |
14 | * <p>(C) DOMOLOGIC Home Automation GmbH 2003-2005</p> |
15 | */ |
16 | public class VoleAnalogMeterExample extends Frame { |
17 | AnalogMeter am1, am2, am3; |
18 | |
19 | /** |
20 | * Create three AnalogMeters with different configurations. |
21 | */ |
22 | public VoleAnalogMeterExample() { |
23 | Container container = new Container(); |
24 | jcontrol.io.Backlight.setBrightness(255); |
25 | // create the first AnalogMeter |
26 | am1 = new AnalogMeter(0, 10, 30, 30, 0, 70, 90, |
27 | AnalogMeter.ORIENTATION_LEFT, 10); |
28 | am1.setNumericDisplay(4, 1, null); |
29 | |
30 | container.add(am1); |
31 | container.add(new Label("RPM", 0, 42, 30, 10, |
32 | Label.ALIGN_CENTER)); |
33 | |
34 | // create the second AnalogMeter |
35 | am2 = new AnalogMeter(35, 10, 58, 30, 0, 120, 180, |
36 | AnalogMeter.ORIENTATION_CENTER, 20); |
37 | am2.setNumericDisplay(4, 1, null); |
38 | |
39 | container.add(am2); |
40 | container.add(new Label("Volt", 35, 42, 58, 10, |
41 | Label.ALIGN_CENTER)); |
42 | |
43 | // create the third AnalogMeter |
44 | am3 = new AnalogMeter(100, 10, 30, 30, 0, 200, 90, |
45 | AnalogMeter.ORIENTATION_RIGHT, 10); |
46 | am3.setNumericDisplay(4, 2, null); |
47 | |
48 | container.add(am3); |
49 | container.add(new Label("Ampere", 95, 42, 30, 10, |
50 | Label.ALIGN_CENTER)); |
51 | |
52 | // add a border |
53 | container.add(new Border("AnalogMeters", 0, 0, 128, 51)); |
54 | |
55 | // add the container to the frame |
56 | this.add(container); |
57 | |
58 | // make us visible |
59 | show(); |
60 | |
61 | |
62 | // create some random values |
63 | for (;;) { |
64 | am1.setValue(Math.rnd(70)); |
65 | am2.setValue(Math.rnd(120)); |
66 | am3.setValue(Math.rnd(200)); |
67 | |
68 | try { |
69 | ThreadExt.sleep(1000); |
70 | } catch (InterruptedException e) {} |
71 | } |
72 | } |
73 | |
74 | // Instantiate and show the VoleAnalogMeterExample |
75 | public static void main(String[] args) { |
76 | new VoleAnalogMeterExample(); |
77 | } |
78 | } |
Listing 1: VoleAnalogMeterExample.java
|