| |
|
Static Components
Besides the manifold GUI components that interact with the user, JControl/Vole furthermore provides some static components to display texts, images or graphical layout elements.
|
Label
The class Label represents a GUI component, which can display a String or an image resource. Different horizontal alignments (left, centered and right) may thereby be specified. The Label autonomously repaints its contained object if it has been overlapped by another GUI component. Label s allow to comfortably place and align texts and images without major programming effort. The most important methods of Label are shown in table 7.
Method | Description |
Label(Object label, int x, int y) | This constructor fast creates a new Label at the desired position. Parameter label can be either an image resource (jcontrol.io.Resource ) or a String . The components' dimensions will be automatically calculated, according to the passed resource. |
Label(Object label, int x, int y, int width, int height, int align) | Creates a new Label at the desired position and with the given dimensions. The parameter align controls the Label s' alignment and will adopt either of the following values: ALIGN_LEFT , ALIGN_RIGHT or ALIGN_CENTER . |
Label(String label, int x, int y, int align) | Special Label constructor which will display a left aligned String at the given coordinates. |
setAlign(int align, boolean revalidate) | Readjusts the horizontal alignment of the Label s' content. If revalidate is true , the area occupied by the Label will be cleared before it is repainted. |
setLabel(Object label, boolean revalidate) | Changes the content of the Label . revalidate may be used to force a recalculation of the preferred component size. |
Table 7: Methods of class jcontrol.ui.vole.Label
Border
Class Border , which has already been used in previous program examples, can be used to draw different types of borders to the display. The borders are transparent and therefore do not occlude already existing GUI components. An optional String can be passed on construction, which will be displayed left aligned in the upper edge of the Border . Like the Label component, Border will automatically repainted if it has been occluded by another component.
Methods | Description |
Border(int x, int y, int width, int height) | Creates a new Border at the desired position and with the given dimensions. |
Border(String label, int x, int y, int width, int height) | Creates a new Border at the desired position, with the given dimensions and the specified label . |
setLabel(String label) | Changes the Border s' label . |
setStyle(int m_style) | Changes the Border s' style to m_style . Possible values are SIMPLE_BORDER (standard), ETCHED_BORDER (emphasised edges) and ROUND_BORDER (round corners). |
Table 8: Methods of class jcontrol.ui.vole.Border
The program example VoleLabelBorderExample.java demonstrates the usage of Label and Border . It is contained in the archive VoleLabelBorderExample.zip along with an according JControl/IDE project file. Figure 5 shows a screenshot.
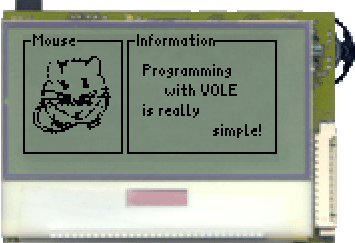
Figure 5: The VoleLabelBorderExample
1 | import java.io.IOException; |
2 | |
3 | import jcontrol.io.Resource; |
4 | import jcontrol.ui.vole.Border; |
5 | import jcontrol.ui.vole.Frame; |
6 | import jcontrol.ui.vole.Label; |
7 | |
8 | /** |
9 | * <p>This example demonstrates how to use the |
10 | * components List and TextArea within the GUI |
11 | * framework JControl/Vole. |
12 | * This program needs the image resource |
13 | * 'mouse.jcif'.</p> |
14 | * |
15 | * <p>(C) DOMOLOGIC Home Automation GmbH 2003-2005</p> |
16 | */ |
17 | public class VoleLabelBorderExample extends Frame { |
18 | Label imageLabel; |
19 | |
20 | /** |
21 | * Create a few Labels and Borders. |
22 | */ |
23 | public VoleLabelBorderExample() { |
24 | |
25 | // create an image Label |
26 | try { |
27 | Resource img = new Resource("mouse.jcif"); |
28 | imageLabel = new Label(img, 5, 10); |
29 | } catch (IOException e) {} |
30 | |
31 | Border b = new Border("Mouse", 0, 0, 50, 60); |
32 | |
33 | // add mouse and border to the frame |
34 | this.add(imageLabel); |
35 | this.add(b); |
36 | |
37 | // now create some text labels with different alignments |
38 | this.add(new Label("Programming", 60, 15)); |
39 | this.add(new Label("with VOLE", 60, 25, 60, 10, |
40 | Label.ALIGN_CENTER)); |
41 | this.add(new Label("is really", 60, 35)); |
42 | this.add(new Label("simple!", 60, 45, 60, 10, |
43 | Label.ALIGN_RIGHT)); |
44 | |
45 | // at last, add a 2nd border |
46 | this.add(new Border("Information", 52, 0, 75, 60)); |
47 | } |
48 | |
49 | |
50 | /** |
51 | * Instantiate and show the VoleLabelBorderExample |
52 | */ |
53 | public static void main(String[] args) { |
54 | new VoleLabelBorderExample().show(); |
55 | } |
56 | } |
Listing 5: VoleLabelBorderExample.java
|