ComboBox und NumberChooser
ComboBox
The ComboBox
is an interactive GUI component, that presents the user multiple text items to choose from. While in inactive state, it is closed and only shows the currently selected item. When it gets the input focus, the ComboBox can be opened by using the Select function. The user may now choose one of the other provided items. If the list of possible choices is bigger than the viewable area, it will be srolled up or down.
The most important methods of the class jcontrol.ui.vole.ComboBox
are shown in table 1:
Method | Description |
ComboBox(int x, int y) | Creates a new ComboBox at the given coordinates |
add(String item) | Adds a new entry to the ComboBox |
String getSelectedItem() | Returns the currently selected entry of the ComboBox as a String |
int getSelectedIndex() | Returns the index of the currently selected entry of the ComboBox as an int |
remove(String item) | Removes an item from the ComboBox |
select(int index) | Selects the {index}th entry of the ComboBox . |
Table 1: Methods of class jcontrol.ui.vole.Container
Installing an ActionListener
onto a ComboBox
(using method setActionListener(ActionListener listener)
) will result in the ComboBox
firing ITEM_SELECTED
-ActionEvents every time the user selects an item.
NumberChooser
The NumberChooser
is a GUI component consisting of a text field and two buttons. The text field displays a decimal value that can be altered using either of the buttons. The value will be in- or decreased by 1 by pressing the according button (the step size can't be varied directly). Minimal and maximal values are to be assigned by using the constructor. Font as well as size and position of the GUI component can be influenced by the programmer. The following methods are provided:
Method | Discription |
NumberChooser(int x, int y, int min, int max) | Creates a new NumberChooser at the given position with a values margin defined by the parameters min and max . |
int getValue() | Returns the current value of the NumberChooser component |
setFont(Resource font) | Changes the components' font into the passed font |
setValue(int value) | Sets the value of the NumberChooser to the passed value (the value has to be within the defined values margin) |
Table 2: Methods of class jcontrol.ui.vole.NumberChooser
A short source code shall be given at the conclusion of this section, that demonstrates the usage of the GUI components ComboBox
and NumberChooser
. It is also available in the archive VoleComboNumberExample.zip. Figure 2 shows a screenshot of the running program.
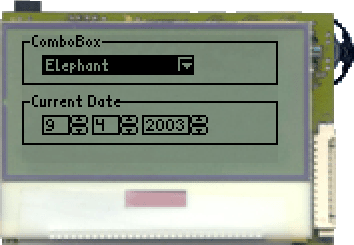
Figure 2: The VoleComboNumberExample
1 | import jcontrol.system.Time; |
2 | import jcontrol.ui.vole.Border; |
3 | import jcontrol.ui.vole.ComboBox; |
4 | import jcontrol.ui.vole.Container; |
5 | import jcontrol.ui.vole.Frame; |
6 | import jcontrol.ui.vole.NumberChooser; |
7 | |
8 | /** |
9 | * <p>This example demonstrates how to use the |
10 | * components ComboBox and NumberChooser within |
11 | * the GUI framework JControl/Vole.</p> |
12 | * |
13 | * <p>(C) DOMOLOGIC Home Automation GmbH 2003-2005</p> |
14 | */ |
15 | public class VoleComboNumberExample extends Frame { |
16 | |
17 | /** |
18 | * Create Vole ComboBox and NumberChooser UI elements. |
19 | */ |
20 | public VoleComboNumberExample() { |
21 | // create a new ComboBox |
22 | ComboBox cb = new ComboBox(10,10); |
23 | |
24 | // add some entries |
25 | cb.add("Vole"); |
26 | cb.add("Mole"); |
27 | cb.add("Tiger"); |
28 | cb.add("Lion"); |
29 | cb.add("Elephant"); |
30 | cb.add("Tyrannosaurus Rex"); |
31 | |
32 | // add the box to our Frame |
33 | this.add(cb); |
34 | |
35 | |
36 | // create some NumberChoosers and a Container to hold them |
37 | Container c = new Container(); |
38 | |
39 | NumberChooser nc1 = new NumberChooser(10,40,1,31); |
40 | NumberChooser nc2 = new NumberChooser(35,40,1,12); |
41 | NumberChooser nc3 = new NumberChooser(60,40,2000,2099); |
42 | |
43 | // set current date |
44 | Time t = new Time(); |
45 | nc1.setValue(t.day); |
46 | nc2.setValue(t.month); |
47 | nc3.setValue(t.year); |
48 | |
49 | // add the NumberChoosers to the Container |
50 | c.add(nc1); |
51 | c.add(nc2); |
52 | c.add(nc3); |
53 | |
54 | // add the Container to the frame |
55 | this.add(c); |
56 | |
57 | |
58 | // nice borders |
59 | this.add(new Border("ComboBox", 0,0,128,25)); |
60 | this.add(new Border("Current Date", 0,30,128,25)); |
61 | } |
62 | |
63 | |
64 | public static void main(String[] args) { |
65 | new VoleComboNumberExample().show(); |
66 | } |
67 | } |
Listing 2: VoleComboNumberExample.java