MenuBar
The MenuBar
is a horizontal line with text-based entries and scroll capability. Due to its small dimensions, it is especially suited for application programs, in which the menu is intended be visible all the time. A special constructor parameter position
allows the automatic alignment at the upper or lower edge of the screen (ALIGN_TOP
and ALIGN_BOTTOM
respectively). Individual fonts might be used by invoking the method setFont(Resource font)
.
The following program example VoleMenuBarExample
creates a menu of the type MenuBar
at the lower edge of the screen and draws it. To try the program, download the archive VoleMenuBarExample.zip and open the contained project file VoleMenuBarExample.jcp
in your JControl/IDE.
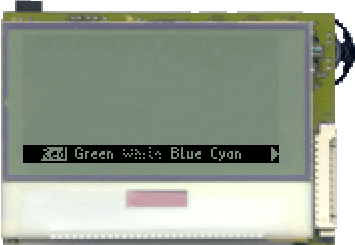
Figure 2: The VoleMenuBarExample
1 | import jcontrol.ui.vole.Frame; |
2 | import jcontrol.ui.vole.menu.MenuBar; |
3 | |
4 | /** |
5 | * <p>This example demonstrates how to use the |
6 | * component MenuBar within the GUI framework |
7 | * JControl/Vole.</p> |
8 | * |
9 | * <p>(C) DOMOLOGIC Home Automation GmbH 2003-2005</p> |
10 | */ |
11 | public class VoleMenuBarExample extends Frame { |
12 | |
13 | /** |
14 | * Create and show a MenuBar. |
15 | */ |
16 | public VoleMenuBarExample() { |
17 | |
18 | // create the MenuBar |
19 | MenuBar menu = new MenuBar(0, 0, 128, 64, MenuBar.ALIGN_BOTTOM); |
20 | |
21 | // add some menu items |
22 | menu.addMenuItem("Red"); |
23 | menu.addMenuItem("Green"); |
24 | menu.addMenuItem("White"); |
25 | menu.addMenuItem("Blue"); |
26 | menu.addMenuItem("Cyan"); |
27 | menu.addMenuItem("Black"); |
28 | menu.addMenuItem("Gray"); |
29 | menu.addMenuItem("Orange"); |
30 | |
31 | // add the menu bar to the Frame |
32 | this.setMenu(menu); |
33 | |
34 | // disable a menu item |
35 | menu.enableMenuItem("White", false); |
36 | |
37 | // show the frame |
38 | setVisible(true); |
39 | } |
40 | |
41 | /** |
42 | * Instantiate the VoleMenuBarExample |
43 | */ |
44 | public static void main(String[] args) { |
45 | new VoleMenuBarExample(); |
46 | } |
47 | } |
Listing 1: VoleMenuBarExample.java